Using animate.css
Now that we have created a transition manually, let’s make things a bit simpler for ourselves by making use of the great open source animate.css
library, which comes with many interesting animations that we can easily use in our angularjs application. You can find out more about this library and the source code at https://github.com/daneden/animate.css. We install animate.css
by running the following in the command line:
bower install --save animate.css
Next, we modify index.html
to load animate.css
:
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, user-scalable=no">
<title>Angular Animation</title>
<link rel="stylesheet" href="bower_components/bootstrap/dist/css/bootstrap.css">
<link rel="stylesheet" href="bower_components/animate.css/animate.css">
<link rel="stylesheet/less" type="text/css" href="css/main.less">
<script src="bower_components/less/dist/less-1.5.1.js"></script>
</head>
With these modifications in place, we can now edit our main.less
file, which will become significantly simpler, despite the fact that we are now also introducing prefixed versions for legacy Mozilla and Microsoft browsers:
body { margin: 100px 40px; font-family: sans-serif; } @enter: 1s; @leave: 1s; .item { width: 230px; padding: 10px; margin: 10px 0; background: #ddd; box-shadow: 5px 5px 5px #888888; font-weight: bold; position: relative; } .item.ng-enter { -webkit-animation: lightSpeedIn @enter; -moz-animation: lightSpeedIn @enter; -ms-animation: lightSpeedIn @enter; animation: lightSpeedIn @enter; } .item.ng-leave { -webkit-animation: lightSpeedOut @leave; -moz-animation: lightSpeedOut @leave; -ms-animation: lightSpeedOut @leave; animation: lightSpeedOut @leave; }
Note that all we really need to do is call the named animations from animate.css
inside the ng-enter
and ng-leave
classes. This is how the result looks when checking off an item:
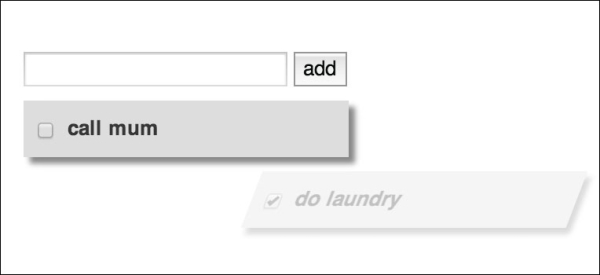
Pretty good, particularly considering that all we had to do was to import a library. There are all kinds of great animations available in
animate.css
, and the source code is available too, making it a great way to learn how these animations are actually created with CSS. CSS animations are a mighty feature, and we can only scratch the surface here.