angularjs 1.2 or higher introduced a new feature in its ngAnimate
module, that is, a native staggering support to CSS transitions and keyframe animations without much CSS code from our end. The built-in $animate
service does all the Grunt work to render animations in a staggering fashion. To get more clarity on this, let’s take a look at the following example.
Let’s modify the addItem()
method from controllers.js
to add multiple to-dos at the same time with a comma as the separator. We’ll then split the inputText
object by a comma to add each to-do separately:
$scope.addItem = function () { var arrTodos = $scope.inputText.split(','); for(var i = 0; i < arrTodos.length; i++) { $scope.items.push({ text: arrTodos[i], completed: false }); } $scope.inputText = ""; };
With this change, we want to see how multiple to-do items are animated simultaneously. If you’ve done said modifications, then you’ll see all the to-dos move at the same time in the following screenshot, which looks quite weird:
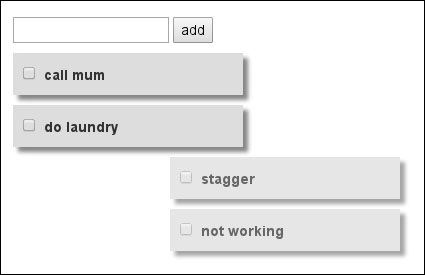
What if we could issue a slight delay in between that would bring a curtain-like effect to the animation? This is what staggering animations do. They allow us to add a delay while animating multiple elements.The stagger effect can be performed by creating a
ng-EVENT-stagger
CSS class and attaching it to the base class used for the animation. The style
property expected within the stagger
class can be a transition-delay
or an animation-delay
property (or both, depending on your requirement). We’ll extend the example we saw in the earlier section, Using LESS to scale entire animations, by adding staggering
classes in main.less
, as follows:
.item.ng-enter-stagger,.item.ng-leave-stagger,.item.ng-move-stagger {-webkit-transition-delay: 0.1s; transition-delay: 0.1s; -webkit-transition-duration:0; transition-duration:0; }
Note that a 100 ms delay will be used between each successive enter/leave/move operation. The last two lines are pretty much required to prevent any kind of CSS inheritance issues, as the chances are that the transition-duration
property is being accidentally inherited and, therefore, the stagger may not work as expected. Here is how the stagger animation will look:
Understanding how staggering works
Well, it’s fairly simple, but it is complex under the hood. The ngAnimate
service looks for the ng-EVENT-stagger
CSS class associated with the element and extracts the animation/transition details to perform the staggering effect. It also takes care of when the animation starts, ends, and how much delay was proposed for a stagger operation so that each animation is spaced out. Note that the service is only there to parse the timing details in order to delay the addition/removal of the ng-*
classes accordingly.]