A QR code is a type of barcode that can be read easily by a digital device that stores information as a series of pixels in a square-shaped grid.
In this modern technological era, QR code is very essential. All modern technology provides QR code-generating process.
Today we are going to learn, how to generate QR codes in laravel with examples.
After finishing this tutorial, you’ll learn how to generate and control the size, color, background color of a QR code. So, let’s start.
Prerequisite :
To complete this tutorial all you need to have is a configured computer, a text editor, a web browser, a web server installed on your computers like Xampp or WampServer, composer(package manager), and a basic understanding of PHP and laravel.
In this tutorial, we will use Sublime Text 3 as a text editor, xampp server, and google chrome as a web browser.
Steps To Generate A QR Code In Laravel
- Download Laravel 10 application
- Install QR code package
- Create Controller
- Create Route
- Create Blade View
- Run Development Server
- Customization
- Advantages of QR code
Download Laravel 10 application
In order to generate a QR code, we need to download and set up a laravel application.
With Composer installed on your computer, you can download a laravel project with the following command-
composer create-project laravel/laravel laravel-qrcode-generator
Install the QR code package
You need to install an external package called simple-qrcode to generate a QR code. You can install it with the following command by typing on your command prompt-
composer require simplesoftwareio/simple-qrcode
Create Controller
For this project, we need to create a controller, so that we can generate a blade view to show the QR code. The controller is a class where we handle requests, and implement logic.
In your laravel project, they are stored in the app/Http/Controllers directory. With this php artisan command, you can make a controller-
php artisan make:controller QrcodeController
In this example, we’re going to use the name QrcodeController. Inside the controller, you’ll need just one function to view the data-
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class QrcodeController extends Controller
{
public function view(){
return view('view_qr_code');
}
}
In this controller, the view() function returns a blade file called “view_qr_code”.
Create Route
Now the package is installed, and the controller is created, you need to create a route. In your installed laravel project there is a file called web.php. Routes are written in that file. Like this-
Route::get('/qr-code',[App\Http\Controllers\QrcodeController::class,'index'])->name('add-qr-code');
Create Blade View
Now we’ve our routes, controller, and the simple-qr code package installed, you need to create a blade file like “view_qr_code” for writing the HTML codes. For this project, the code is given below-
<h1>How to generate QR code in Laravel</h1>
<div class="container">
<!-- generate function from simple-qr code package -->
{{QrCode::generate('Hello!');}}
</div>
<div class="codesource-link">
<a href="https://https://codesource.io/">Codesource</a>
</div>
Here in this HTML code, we simply created a HTML and call the generate() function from the simple-qrcode package and put it inside double curly braces to print the generated QR code.
Run Development Server
Now run the development server with the following PHP artisan command and see what happens-
php artisan serve
OUTPUT:

As you can see, it is very easy to generate a QR code. Also, you can customize it as you want.
Customization
Increasing the size:
You can increase the size of the QR code as you want-
{{QrCode::size(200)->generate('Hello!');}}
You need to specify the size inside the parameter of the size() function to control the size of the QR code.
Output:
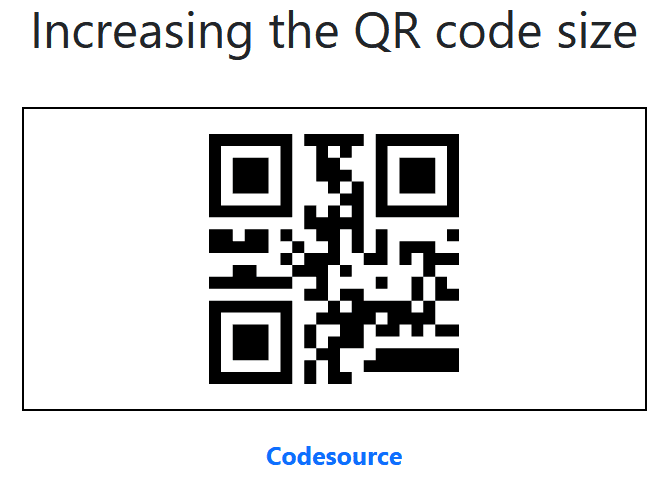
Changing the color:
You can change the color also with the color() function. Inside the function parameter, you’ve to give the RGB color codes.-
{{QrCode::color(0,100,0)->generate('Hello!');}}
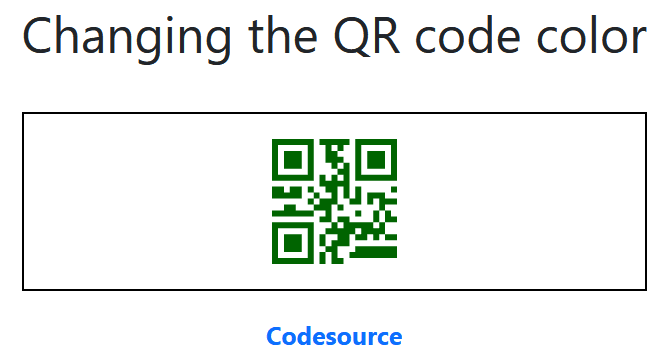
Changing the background color:
Also, you can change the background color of the QR code. Like this-
{{QrCode::backgroundColor(0,100,0)->generate('Hello!');}}
Output:
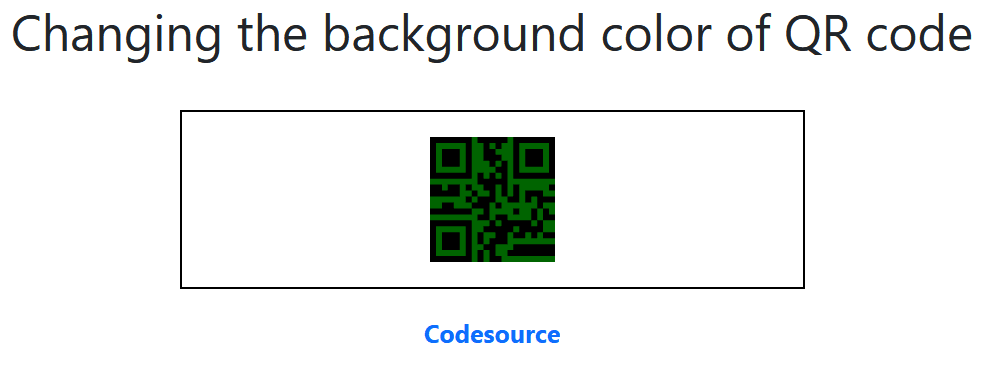
Advantages of QR code:
As a user, you get instant access to more information about a product or service directly from your mobile.
You don’t have to type anything into your mobile, by just using the camera and scanning the QR code, all information stored in the QR code is available via your mobile phone.
Conclusion:
In this tutorial, we learned how to generate a QR code in laravel and completed a small project. To use it better, you’ve to practice it more. Take this tutorial as a reference and it may help you to achieve your goal. Good luck.