In this article, I will explain how to do server-side pagination in Angular apps using ngx-pagination.
Server-side pagination is returning a subset of data requested by a client if you don’t want to display all data at once.
The server takes a certain parameter from the client to get relevant information/records.
ngx-pagination
ngx-pagination is a simple package(solution) for pagination in angular. It allows paging (displaying a subset of data by page).
Getting started with ngx-pagination.
Installing the package is as simple as running
npm install ngx-pagination --save
After installation, import NgxPaginationModule
to your app module or any module you’ll like to use the package. See the example below;
// app.module.ts
import {NgxPaginationModule} from 'ngx-pagination'; // <-- import the module
@NgModule({
imports: [
NgxPaginationModule // <-- include it in your app module
],
})
In the component file, add the following code
collection: any;
p: number;
constructor( private http: HttpClient) {}
ngOnInit() {
this.getAllData();
}
getAllData() {
const url = "https://api.instantwebtools.net/v1/passenger";
this.http.get(url).subscribe((data: any) => {
console.log(data);
this.collection = data;
})
};
}
In the code snippet above I defined a collection property of type any, likewise a ‘p’ property of type number.
line 3:, I created an instance of Angular HttpClient Library, so I can use it to communicate with the API as used on line 11.
from line 9, I’m calling the getAllData()
method and subscribing to it. Once the data is received, I am binding it to the collection property object and also console.logging
it.
The getAllData()
function is placed inside of ngOnInit()
function, which is a lifecycle hook for Angular. Any code placed in here will run when the component is loaded
In the component file, add the following code
Go into your template file to use its paginate pipe and its paginationControlComponent like so.
<ul>
<li *ngFor="let item of collection.data | paginate: { itemsPerPage: 10, currentPage: p }"> </li>
</ul>
<pagination-controls (pageChange)="p = $event"></pagination-controls>
The above code will generate the image
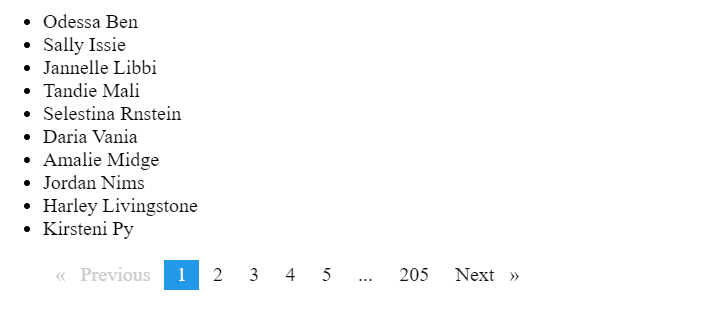
Please note, the above was made possible with dummy API from Instant Webtools.
Now that we have the data populate on the view and been controlled with ngx-pagination, let’s take a look at the console to see how much data was called at once.
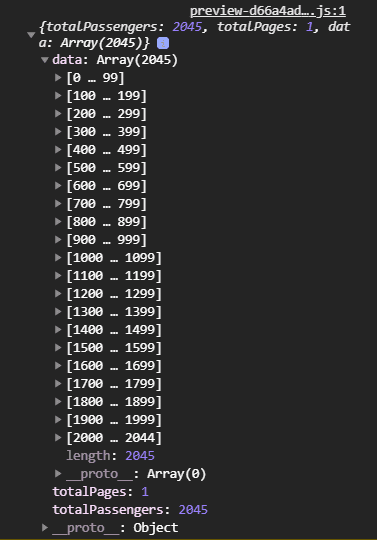
OMG!!! ? this is a whole lot of data been fetched at once.
This is where server-side pagination comes in.
Let’s change our code to call 5 data per page from the server instead of the whole data.
This scenario is supported by ngx-pagination by using the totalItems config option.
This will allow the correct number of page links to be calculated.
Refactored code below
collection: any;
p: number;
itemsPerPage = 5;
totalItems: any;
constructor( private http: HttpClient) {}
ngOnInit() {
this.getAllData();
}
getAllData() {
const url = `https://api.instantwebtools.net/v1/passenger?page=${0}&size=${this.itemsPerPage}`;
this.http.get(url).subscribe((data: any) => {
console.log(data);
this.collection = data;
this.totalItems = data.totalPassengers;
})
}
getPage(page) {
const url = `https://api.instantwebtools.net/v1/passenger?page=${page}&size=${this.itemsPerPage}`;
this.http.get(url).subscribe((data: any) => {
console.log(data);
this.collection = data;
this.totalItems = data.totalPassengers;
})
}
<ul>
<li *ngFor="let item of collection.data | paginate: { itemsPerPage: itemsPerPage , currentPage: p, totalItems: totalItems }"> {{item.name}} </li>
</ul>
<pagination-controls (pageChange)="getPage(p = $event)"></pagination-controls>
This is an improvement on the above code. The first API request returns all post at once but this (line 12 and line 21 ) allows requesting a paged request.
Line 3: This is a property that use on line 12 and 21 to specify items to show per page(5).
Line 4: I created another property called totalItems
as specified by ngx-pagination.
Line 16: I assigned the totalPassenger
from the data to totalItems
which was created on line 4.
Line 20 – 28: getPage() is a function/method from the html code which takes page parameter.
It performs the same function as getAllData()
function but this time, it is controlled by the argument(page) it receives.
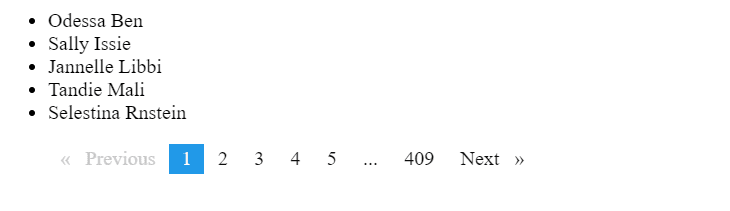
Let’s now check our console/network tab to see the total request per page.
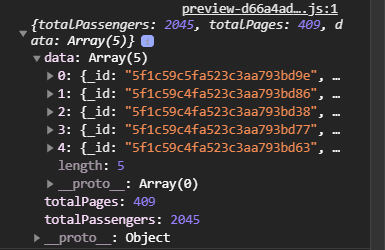
We now get 5 requests per page. This will aid our app response time in some ways.
The complete code for this article can be found here.
The above code is for learning purposes only. The HTTP request could be done from a service file instead. Angular has good ways of structuring projects.
Resources