In this tutorial, you will learn How to read and write files in NodeJS.
NodeJS is very fast and highly scalable because of its event-driven, single-threaded, and, non-blocking I/O model and you can do many interesting things by using it.
Reading and writing files is one of them.
It is a very essential functionality that is provided by NodeJS and the implementation of these things is very easy.
You can read or write a file from your computer both synchronously or asynchronously.
We will cover both of these in this tutorial and after completing this tutorial you will be able to read and write your own file from the computer both in a synchronous and asynchronous way.
Steps to Read and write files in NodeJS
- Understand NodeJS
‘fs’
module - Reading file Synchronously
- Writing file Synchronously
- Reading file Asynchronously
- Writing file Asynchronously
- Error handling
What is NodeJS ‘fs’ module
NodeJS lets you perform different useful actions with its built-in modules. fs module is one of them. The meaning of fs is a file system that helps you to work with files. such as read file, write file, append file, and so on. It will work both in a synchronous and asynchronous way. Let’s see the below code example of how you can import the fs module in your project.
const fs = require('fs')
Here, we have used const
instead of using var and it is an ES6 feature. It will require the module from NodeJS built-in module and is available for you to use it. We will import this module to work with the file system and read and write our file.
Reading file Synchronously
We will read a file from our local file. In this section, we will read a file synchronously, and to do so we will use fs.readFileSync()
function. We already know about the fs module and this module helps us to read a file synchronously. It takes two arguments, the first one is the path of the particular file that will be read and the next one is the character encoding. If you don’t use the character encoding then the output will be shown as a buffer. Let’s see the below code example:
const fs = require('fs')
const textRead = fs.readFileSync('./text/readfile.txt','utf-8')
console.log(textRead)
Here, we import the fs module from NodeJS built-in module and then read a file that is located in the same directory in the text folder with the name of readfile.txt. This readFilySync()
` function will return the file that we have mentioned. So we save the return file into textRead variable and finally print it to the console. If everything is fine then we will be able to see the output with some text that we have written in the file. Let’s see the output below:

You can see that our file has been read successfully in the console and to see the output we give the command in our console which is node app.js. In your case, all you need to do is to type node, and then your file name in the console.
Writing file Synchronously
We will write a file externally and save it in our directory. It will follow the same process that we have followed in the reading file section except to write a file we will use fs.writeFileSync()
function and in this function, we will define a particular path where the file will be saved and for our easiness, we will define a variable where we will write some text. Let’s see the file structure first:
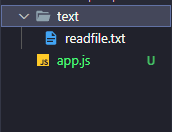
Here, you can see that in our text folder we have only one file named readfile. Let’s write a file with the help of NodeJS and see if there any changes occurred or not in the below:
const fs = require('fs')
const txtWrite = `This is a write file and it will save this text into text folder\\n`
fs.writeFileSync('./text/writefile.txt', txtWrite)
console.log('File has been written')
Here, we take a variable and write some text and then save this file. The fs.writeFileSync() function does not return anything and that’s why we didn’t use any variable and finally, we put a console log with a message that the file has been written. Let’s see the console first:

In our console, we can see that the message has been showing successfully. Now let’s check the text folder is there any changes that happened or not.
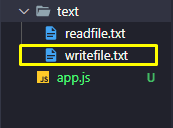
You can see that a new file has been created named writefile.txt right under the readfile.txt. That means we are successfully written a file by using NodeJS and let’s see if the texts exist or not in the writefile.txt

Here, the text that we have written is saved perfectly into the writefile.txt file. This is how you can read and write files synchronously by using NodeJS. Let’s see how you can read and write files asynchronously in the next few sections.
Reading file Asynchronously
We will read our file asynchronously by using fs.readFile()
function. It is non-blocking which means it does not block the rest of the code while executing.
I assume that you already know about basic JavaScript functions and this asynchronous function is a feature of JavaScript.
This readFile()
function expected two arguments where we will define our path and character encoded and it also calls a callback function with two arguments one is the error and another is the data.
If any error occurred it will show an error otherwise it will return the particular data. We will handle the error later but first, let’s see a basic example of reading files asynchronously below :
const fs = require('fs')
fs.readFile('./text/readFileAsync.txt','utf-8', (err, data) => {
console.log(data)
})
console.log('File has been reading Asynchronouly')
Here, we almost do the same but in an asynchronous way, and finally, we console log a simple message just to demonstrate to you that it is working in a non-blocking manner. Let’s see the output below:

You can see that the message was showing first and then reading the file. The reason behind it is we have used an asynchronous function as a result it didn’t block other codes while executing itself.
Writing file Asynchronously
To write a file in an asynchronous way we need to use fs.writeFile()
function. It also works the same as writeFileSync()
function except it returns a callback function.
In this case, the callback()
function only expects one argument for error handling. Let’s write a file by using an asynchronous function in the below:
const fs = require('fs')
const data = 'This is a text file and it will be written asynchronously by using NodeJS\\n'
fs.writeFile('./text/writeFileAsync.txt',data,'utf-8',(err)=>{})
console.log('File has been written Asynchronouly')
Here, we have taken a variable named data and written some text inside it, and finally save it into our local computer by using fs.writeFile()
function and finally print a message in the console just to inform that our file has been written. Let’s see the console first:

You can see that our message has been shown successfully in the console. Let’s see our file has been written with particular data or not in the below:

Here, our file has been created successfully with the particular text that we have given before. This is how you can read and write files asynchronously by using NodeJS. Let’s see the use of use arguments in the below section.
Error handling
Error handling is important and essential for developers. To find an error and debug it is of great quality. We have seen that an err
argument exists while working on reading and writing files asynchronously but we did not handle it. Let’s see a quick overview of how to use it below:
const fs = require('fs')
fs.readFile('./text/readFileAsyncccc.txt','utf-8', (err, data) => {
if (err) return console.log(err)
console.log(data)
})
we know that in our file directory, there is no file that exists named readFileAsyncccc.txt so it will simply give an error and we will track down the error with a beautiful error message and description of the error.
If there is no error then data will print in the console and if there’s any then it will return the error and block other code from being executed inside the function.
We know that in that function there’s an error occurred let’s run the program with the node command and see what happens below:

You can see that there’s an error occurred and instead of printing the data it shows an error message in the console this is how you can handle errors while reading and writing files asynchronously.
Conclusion
This is all about reading and writing files in NodeJS. Now you know almost everything about how to read and write a file in NodeJS both in a synchronous and asynchronous way. We have coved about NodeJS built-in fs module system and also the error handler and use of synchronous and asynchronous functions while working with the file system. These things will help you with your future journey of NodeJS. All you need to do is to take this tutorial as a reference and try to learn and implement new things.