React Native PrismJs is a real thing. With the help of WebView
, it is quite possible. Here in this article, we will learn about the ways in which PrismJs could be integrated with React Native.
Introduction
PrismJs is the library written in JavaScript, which prettifies your <code>
markups like IDEs. It supports nearly all the major languages in the market. Generally we use it for the websites only, but in this article I am going to show you how it could be used in mobile apps as well. I will also show you a working example.
Before You Begin
This is not an article to learn React Native or PrismJs or WebView. I have created it to show you the way to integrate Prismjs with React Native. So, you need to have a little idea of all the 3 components and Html.
Step 1: Importing WebView
React Native has deprecated their core WebView (Read More). They are now recommending to use community package instead, like many other components. So, we will need to install it from here
yarn add react-native-webview
If you are running React Native version > 0.60, then there is no need to manually link it but if not then you may read the linking instructions from here
After installing the package, we can simply import it using this command –
import { WebView } from 'react-native-webview';
Step 2: Getting ready with PrismJs
PrismJs provides you Stylesheets and JavaScript files. But they are separate for different languages. Which of them to use, depends on your use case.
Suppose you are adding a python code block in your website then you will need the python js file of Prism. There is whole bunch of supported language to choose from.
For this tutorial, I will let Prism decide by using autoLoader file.
You will be happy to know that Prism files are uploaded on major Cdn like jsDelivr, cdnjs and Unpkg. I will use cdnjs in this article. These are the files we will require –
- https://cdnjs.cloudflare.com/ajax/libs/prism/1.22.0/themes/prism-dark.min.css
- https://cdnjs.cloudflare.com/ajax/libs/prism/1.22.0/components/prism-core.min.js
- https://cdnjs.cloudflare.com/ajax/libs/prism/1.22.0/plugins/autoloader/prism-autoloader.min.js
One more thing here, it works when it sees the <code>
block. So, whatever code you are planning to prettify that should be inside code block and you may assign a language-xxxx
class. For example, if I am writing a javascript code, I will use class language-js
HTML code using PrismJs
This is the html code we will use in our app.
<!DOCTYPE html>
<html>
<head>
<link href="https://cdnjs.cloudflare.com/ajax/libs/prism/1.22.0/themes/prism-dark.min.css" rel="stylesheet" />
</head>
<body style="font-size: 50px">
<script src="https://cdnjs.cloudflare.com/ajax/libs/prism/1.22.0/components/prism-core.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/prism/1.22.0/plugins/autoloader/prism-autoloader.min.js"></script>
<pre>
<code class="language-js">
const reactNative = true;
const prism = true;
if(reactNative && prism) {
console.log('It Works!');
}
else {
console.log('Sad');
}
</code>
</pre>
</body>
</html>
Step 3: Integrate WebView in React Native with PrismJs
Let’s break this step in 2 parts –
- React native code.
- Webview with prism.
In first part, I will write the code and import webview in it.
import * as React from 'react';
import { View, SafeAreaView } from 'react-native';
import { WebView } from 'react-native-webview';
export default function App() {
return (
<View style={{flex:1}}>
<SafeAreaView />
{/*We Will Put WebView Here*/}
</View>
);
}
In this code I am using View
and SafeAreaView
. Also I am importing WebView
from community package, react-native-webview
.
In second step, I will integrate prism with WebView. Here is the code for it –
<WebView
originWhitelist={['https://cdnjs.cloudflare.com/ajax/libs/prism/1.22.0/themes/prism-dark.min.css', 'https://cdnjs.cloudflare.com/ajax/libs/prism/1.22.0/components/prism-core.min.js', 'https://cdnjs.cloudflare.com/ajax/libs/prism/1.22.0/plugins/autoloader/prism-autoloader.min.js']}
source={{ html: `
<!DOCTYPE html>
<html>
<head>
<link href="https://cdnjs.cloudflare.com/ajax/libs/prism/1.22.0/themes/prism-dark.min.css" rel="stylesheet" />
</head>
<body style="font-size: 50px">
<script src="https://cdnjs.cloudflare.com/ajax/libs/prism/1.22.0/components/prism-core.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/prism/1.22.0/plugins/autoloader/prism-autoloader.min.js"></script>
<pre>
<code class="language-js">
const reactNative = true;
const prism = true;
if(reactNative && prism) {
console.log('It Works!');
}
else {
console.log('Sad');
}
</code>
</pre>
</body>
</html>
` }}
/>
In this code I have included the originWhiteList
property of WebView. This property helps in keeping our code secure from XSS attack. Only whitelist those Urls which you trust. Like I have whitelisted only the cdnjs links. If you want to learn more about originWhiteList, refer here.
Live Demo
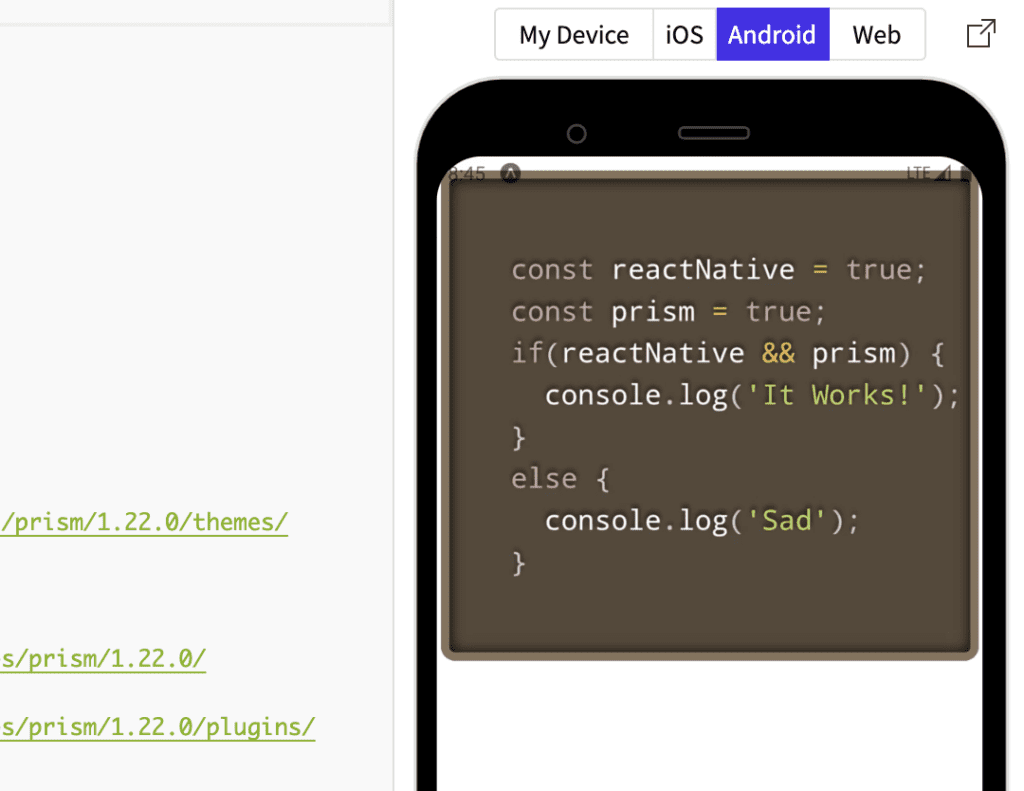
Conclusion
In this article, we have seen how easy it is to use Prism in React Native. It is not necessary to use Html inside webview component. Else, you can also call a URL or store a static HTML file in the app and load that. So, there are a number of ways to work with it.
That’s it for now. See you in the next article. Till then, Happy Coding.